1. 具体需求
克隆羊问题:
现在有一只羊tom,姓名为tom,年龄为1,颜色为白色,请编写程序创建和tom羊属性完全相同的10只羊。
2. 传统解决方式
1)思路分析
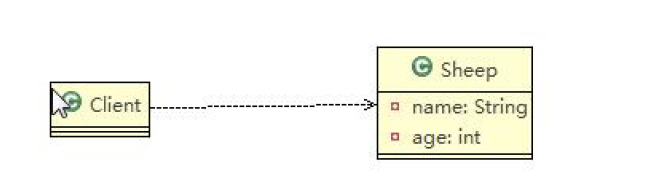
2)代码演示
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34
| public class Sheep { private String name; private int age; private String color; public Sheep(String name, int age, String color) { super(); this.name = name; this.age = age; this.color = color; } public String getName() { return name; } public void setName(String name) { this.name = name; } public int getAge() { return age; } public void setAge(int age) { this.age = age; } public String getColor() { return color; } public void setColor(String color) { this.color = color; } @Override public String toString() { return "Sheep [name=" + name + ", age=" + age + ", color=" + color + "]"; } }
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22
| public class Client {
public static void main(String[] args) { Sheep sheep = new Sheep("tom", 1, "白色"); Sheep sheep2 = new Sheep(sheep.getName(), sheep.getAge(), sheep.getColor()); Sheep sheep3 = new Sheep(sheep.getName(), sheep.getAge(), sheep.getColor()); Sheep sheep4 = new Sheep(sheep.getName(), sheep.getAge(), sheep.getColor()); Sheep sheep5 = new Sheep(sheep.getName(), sheep.getAge(), sheep.getColor()); System.out.println(sheep); System.out.println(sheep2); System.out.println(sheep3); System.out.println(sheep4); System.out.println(sheep5); }
}
|
3)传统方式的优缺点
- 优点是比较好理解,简单易操作
- 缺点是在创建新对象的时候,总是需要重新获取原始对象的属性,如果创建的对象比较复杂时,效率较低
- 总是需要重新初始化对象,而不是动态地获得对象运行时的状态,不够灵活
4)改进思路
Java中Object类是所有类的根类,Object类提供了一个clone()方法,该方法可以将一个Java对象复制一份,但是要实现clone的Java类必须要实现一个接口Cloneable,该接口表示该类能够复制且具有复制的能力->原型模式
3. 原型模式解决方式
3.1 基本介绍
1) 原型模式(Prototype 模式)是指:用原型实例指定创建对象的种类,并且通过拷贝这些原型,创建新的对象 2) 原型模式是一种创建型设计模式,允许一个对象再创建另外一个可定制的对象,无需知道如何创建的细节 3)工作原理是:通过将一个原型对象传给那个要发动创建的对象,这个要发动创建的对象通过请求原型对象拷贝它们自己来实施创建,即对象.clone()
4) 形象的理解:孙大圣拔出猴毛,变出其它孙大圣
3.2 原理图
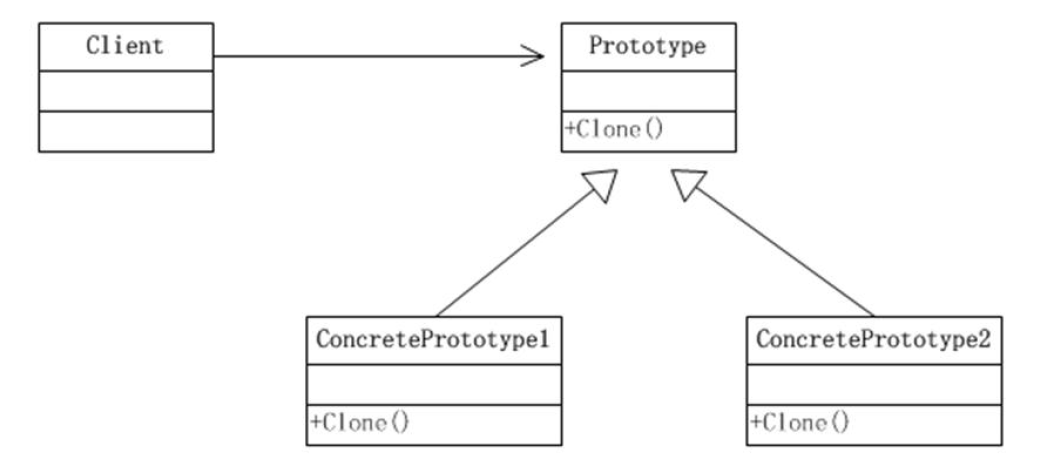
原理图说明:
1)Prototype:原型类,声明一个克隆自己的接口
2)ConcretePrototype:具体的原型类,实现一个克隆自己的操作
3)Clint:客户端,让一个原型对象克隆自己,从而创建一个新的对象(属性一样)
3.3 应用实例
使用原型模式改进传统方式,让程序具有更高的效率和扩展性。
代码实现:
- 写原型类,实现Cloneable,重写clone方法
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46
| public class SheepPrototype implements Cloneable{ private String name; private int age; private String color; public SheepPrototype(String name, int age, String color) { super(); this.name = name; this.age = age; this.color = color; } public String getName() { return name; } public void setName(String name) { this.name = name; } public int getAge() { return age; } public void setAge(int age) { this.age = age; } public String getColor() { return color; } public void setColor(String color) { this.color = color; } @Override public String toString() { return "Sheep [name=" + name + ", age=" + age + ", color=" + color + "]"; }
@Override protected Object clone() { SheepPrototype sheepPrototype = null; try { sheepPrototype = (SheepPrototype)super.clone(); }catch (Exception e){ System.out.println(e.getMessage()); } return sheepPrototype; } }
|
- 使用
1 2 3 4 5 6 7 8 9 10 11 12
| public class Client { public static void main(String[] args) { SheepPrototype sheep = new SheepPrototype("tom", 1, "white"); SheepPrototype sheep2 = (SheepPrototype) sheep.clone(); SheepPrototype sheep3 = (SheepPrototype) sheep.clone(); SheepPrototype sheep4 = (SheepPrototype) sheep.clone(); SheepPrototype sheep5 = (SheepPrototype) sheep.clone(); System.out.println(sheep2.toString()); System.out.println(sheep3.toString()); } }
|
使用的是克隆,两个对象只是属性相同,并不==
4. 原型模式在Spring框架中源码分析
1)Spring中原型bean的创建,就是原型模式的应用
2)代码分析+Debug源码
image-20221125164741778
5. 深入讨论-深拷贝和浅拷贝
5.1 浅拷贝基本介绍
1)对于数据类型是基本数据类型的成员变量,浅拷贝会直接进行值传递,也就是将该属性值复制一份给新的对象。 2) 对于数据类型是引用数据类型的成员变量,比如说成员变量是某个数组、某个类的对象等,那么浅拷贝会进行引用传递,也就是只是将该成员变量的引用值(内存地址)复制一份给新的对象。因为实际上两个对象的该成员变量都指向同一个实例。在这种情况下,在一个对象中修改该成员变量会影响到另一个对象的该成员变量值。(对象的属性没有真正的复制,而是指向同一个空间)
3)前面我们克隆羊就是浅拷贝
4)浅拷贝是使用默认的clone() 方法来实现
sheepPrototype = (SheepPrototype)super.clone();
5.2 深拷贝基本介绍
1)复制对象的所有基本数据类型的成员变量值 2)为所有引用数据类型的成员变量申请存储空间,并复制每个引用数据类型成员变量所引用的对象,直到该对象可达的所有对象。也就是说,对象进行深拷贝要对整个对象(包括对象的引用类型)进行拷贝
- 深拷贝实现方式 1:重写 clone 方法来实现深拷贝
- 深拷贝实现方式2:通过对象序列化实现深拷贝(推荐) ### 5.3 深拷贝应用实例 1)使用重写clone方法实现深拷贝 2)使用序列化来实现深拷贝
- 代码演示
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20
| public class DeepCloneableTarget implements Serializable, Cloneable { private static final long serialVersionUID = 1L;
private String cloneName;
private String cloneClass;
public DeepCloneableTarget(String cloneName, String cloneClass) { this.cloneName = cloneName; this.cloneClass = cloneClass; }
@Override protected Object clone() throws CloneNotSupportedException { return super.clone(); } }
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61
| public class DeepProtoType implements Serializable, Cloneable { public String name; public DeepCloneableTarget deepCloneableTarget; public DeepProtoType() { super(); }
@Override protected Object clone() throws CloneNotSupportedException { Object deep = null; deep = super.clone(); DeepProtoType deepProtoType = (DeepProtoType)deep; deepProtoType.deepCloneableTarget = (DeepCloneableTarget)deepCloneableTarget.clone();
return deepProtoType; }
public Object deepClone() {
ByteArrayOutputStream bos = null; ObjectOutputStream oos = null; ByteArrayInputStream bis = null; ObjectInputStream ois = null;
try {
bos = new ByteArrayOutputStream(); oos = new ObjectOutputStream(bos); oos.writeObject(this);
bis = new ByteArrayInputStream(bos.toByteArray()); ois = new ObjectInputStream(bis); DeepProtoType copyObj = (DeepProtoType)ois.readObject();
return copyObj; } catch (Exception e) { e.printStackTrace(); return null; } finally { try { bos.close(); oos.close(); bis.close(); ois.close(); } catch (Exception e2) { System.out.println(e2.getMessage()); } } } }
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25
| public class DeepClient { public static void main(String[] args) {
DeepProtoType deepProtoType = new DeepProtoType(); deepProtoType.name = "A"; deepProtoType.deepCloneableTarget = new DeepCloneableTarget("Target","ooo");
try { DeepProtoType clone = (DeepProtoType) deepProtoType.clone(); System.out.println("-----方式1-----"); System.out.println("name=" + deepProtoType.name + " deepCloneTarget hashcode = " + deepProtoType.deepCloneableTarget.hashCode()); System.out.println("----After Deep Clone---"); System.out.println("name=" + clone.name + " deepCloneTarget hashcode = " + clone.deepCloneableTarget.hashCode());
DeepProtoType clone2 = (DeepProtoType) deepProtoType.deepClone(); System.out.println("-----方式2-----"); System.out.println("name=" + deepProtoType.name + " deepCloneTarget hashcode = " + deepProtoType.deepCloneableTarget.hashCode()); System.out.println("----After Deep Clone---"); System.out.println("name=" + clone2.name + " deepCloneTarget hashcode = " + clone2.deepCloneableTarget.hashCode()); } catch (CloneNotSupportedException e) { e.printStackTrace(); } } }
|
6. 原型模式注意事项和细节
1)创建新的对象比较复杂时,可以利用原型模式简化对象的创建过程,同时也能够提高效率。 2) 不用重新初始化对象,而是动态地获得对象运行时的状态。 3) 如果原始对象发生变化(增加或者减少属性),其它克隆对象的也会发生相应的变化,无需修改代码。 4) 在实现深克隆的时候可能需要比较复杂的代码 缺点:需要为每一个类配备一个克隆方法,这对全新的类來说不是很难,但对已有的类进行改造时,需要修改其源代码,违背了ocp 原则。